Requesting OAuth Token from AAD Programmatically
Here's a couple options to request an access token from Azure Active Directory programmatically. Note my examples switch between the Azure Commercial and Azure Government endpoints.
Azure CLI Example
token=$(az account get-access-token --resource=https://management.azure.com --query accessToken --output tsv)
curl -X GET --header "Authorization: Bearer $token" https://management.azure.com/subscriptions?api-version=2020-01-01
PowerShell Example
[System.Net.ServicePointManager]::SecurityProtocol = [System.Net.SecurityProtocolType]::Tls12;
$tenantId = "TenantId"
$clientID = "ApplicationId"
$clientSecret = "ClientSecret"
$resource = "https://management.azure.com" #https://management.usgovcloudapi.ent for Azure Government
$authority = "https://login.microsoftonline.com/$tenantId/oauth2/token" #replace .com with .us for Azure Government
$body = @{
"grant_type"="client_credentials" #don’t change this value
"client_id"=$clientId
"resource"=$resource
"client_secret"= $clientSecret
}
$oauth = Invoke-RestMethod -Method Post -Uri $authority -Body $body
$accessToken = $oauth.access_token
$accessToken
$headers = @{
"Authorization"="Bearer $accessToken"
"Content-Type"="application/json"
}
$response = Invoke-RestMethod -Method Get -Uri "https://management.azure.com/subscriptions?api-version=2020-01-01" -Headers $headers #test to get the subscriptions that the Application has access to
$response.value[0]
Postman
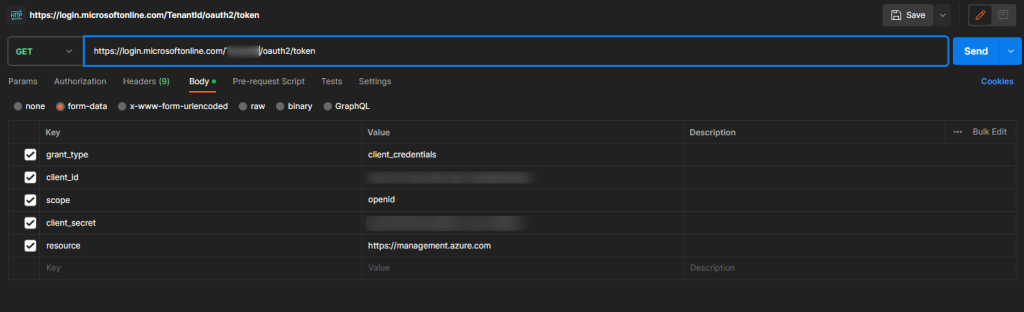
CURL
****Note UNIX doesn't have a good option to parse a json response so either manually copy the value out or use Python on JQ to parse the JSON response.
CLIENTID='Replace_with_client_id'
CLIENTSECRET='Replace_with_secret'
RESOURCE='https://management.core.usgovcloudapi.net'
AUTHORITY='https://login.microsoftonline.us/REPLACE_WITH_TENANT_ID/oauth2/token'
TOKEN=curl -X POST --form 'grant_type=client_credentials' --form 'client_id="'$CLIENTID'"' --form 'Resource="'$RESOURCE'"' --form 'client_secret="'$CLIENTSECRET'"' $AUTHORITY